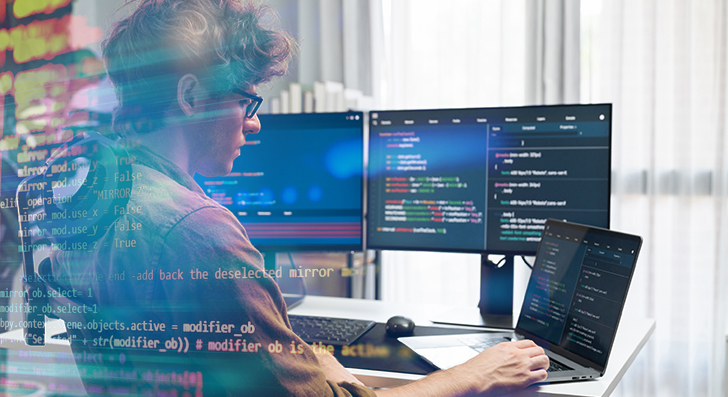
Scalability usually means your application can handle advancement—additional consumers, much more details, plus more website traffic—without breaking. To be a developer, constructing with scalability in mind will save time and tension afterwards. Listed here’s a clear and functional tutorial that can assist you begin by Gustavo Woltmann.
Structure for Scalability from the Start
Scalability is just not anything you bolt on later—it ought to be part of your prepare from the beginning. A lot of applications fall short when they increase fast due to the fact the original structure can’t manage the additional load. As being a developer, you'll want to Believe early about how your procedure will behave under pressure.
Start off by developing your architecture to generally be flexible. Keep away from monolithic codebases where every little thing is tightly related. Instead, use modular design and style or microservices. These styles crack your application into lesser, independent elements. Just about every module or service can scale on its own with no influencing The complete method.
Also, give thought to your database from day a single. Will it need to have to take care of one million users or perhaps a hundred? Select the right kind—relational or NoSQL—depending on how your facts will mature. Approach for sharding, indexing, and backups early, even if you don’t require them but.
One more significant point is to prevent hardcoding assumptions. Don’t create code that only operates beneath recent problems. Contemplate what would materialize In the event your person base doubled tomorrow. Would your application crash? Would the databases decelerate?
Use style and design designs that assist scaling, like concept queues or function-driven methods. These assistance your application cope with additional requests devoid of finding overloaded.
If you Create with scalability in mind, you're not just making ready for success—you're reducing upcoming problems. A properly-planned method is easier to take care of, adapt, and increase. It’s far better to get ready early than to rebuild later.
Use the Right Databases
Picking out the proper database is often a essential Portion of building scalable applications. Not all databases are crafted the exact same, and using the wrong you can slow you down or even bring about failures as your app grows.
Get started by knowledge your facts. Is it really structured, like rows in the table? If Of course, a relational database like PostgreSQL or MySQL is a superb suit. They are solid with relationships, transactions, and consistency. In addition they assistance scaling procedures like go through replicas, indexing, and partitioning to take care of a lot more traffic and knowledge.
If your knowledge is more adaptable—like user exercise logs, product catalogs, or paperwork—consider a NoSQL selection like MongoDB, Cassandra, or DynamoDB. NoSQL databases are better at dealing with substantial volumes of unstructured or semi-structured information and might scale horizontally a lot more conveniently.
Also, contemplate your examine and write designs. Will you be performing a great deal of reads with much less writes? Use caching and read replicas. Have you been managing a hefty publish load? Take a look at databases that may take care of superior write throughput, and even celebration-centered data storage techniques like Apache Kafka (for non permanent information streams).
It’s also wise to Consider in advance. You might not need Sophisticated scaling functions now, but picking a databases that supports them suggests you received’t require to switch later.
Use indexing to speed up queries. Stay away from unneeded joins. Normalize or denormalize your facts determined by your obtain styles. And normally observe database efficiency as you develop.
In brief, the correct database depends upon your app’s structure, speed needs, And exactly how you hope it to develop. Take time to pick sensibly—it’ll help you save many issues later on.
Enhance Code and Queries
Quick code is key to scalability. As your application grows, just about every small hold off provides up. Badly created code or unoptimized queries can slow down general performance and overload your process. That’s why it’s essential to Create effective logic from the start.
Get started by writing cleanse, basic code. Stay away from repeating logic and remove just about anything unneeded. Don’t choose the most complex Option if an easy one is effective. Maintain your features brief, concentrated, and simple to check. Use profiling equipment to locate bottlenecks—sites the place your code requires much too prolonged to run or works by using an excessive amount memory.
Subsequent, evaluate your database queries. These normally sluggish matters down a lot more than the code itself. Be sure each query only asks for the info you actually need to have. Steer clear of Pick out *, which fetches every thing, and as a substitute choose precise fields. Use indexes to speed up lookups. And stay away from accomplishing too many joins, In particular throughout huge tables.
For those who observe the same info staying requested repeatedly, use caching. Retail outlet the results temporarily employing applications like Redis or Memcached so that you don’t really need to repeat highly-priced operations.
Also, batch your databases functions after you can. Rather than updating a row one by one, update them in teams. This cuts down on overhead and would make your application more effective.
Remember to examination with substantial datasets. Code and queries that work wonderful with a hundred documents could possibly crash once they have to deal with 1 million.
In a nutshell, scalable apps are rapidly applications. Maintain your code restricted, your queries lean, and use caching when wanted. These ways assistance your application remain easy and responsive, whilst the load will increase.
Leverage Load Balancing and Caching
As your app grows, it's to deal with a lot more end users and a lot more website traffic. If anything goes by just one server, it'll rapidly become a bottleneck. That’s where load balancing and caching come in. Both of these instruments enable maintain your app quick, stable, and scalable.
Load balancing spreads incoming traffic throughout many servers. In place of a person server accomplishing the many get the job done, the load balancer routes end users to distinct servers according to availability. This means no one server will get overloaded. If 1 server goes down, the load balancer can send visitors to the Other folks. Resources like Nginx, HAProxy, or cloud-based alternatives from AWS and Google Cloud make this straightforward to put in place.
Caching is about storing info temporarily so it could be reused swiftly. When users ask for the identical information all over again—like a product page or maybe a profile—you don’t must fetch it from the databases whenever. You are able to provide it from your cache.
There's two widespread kinds of caching:
one. Server-side caching (like Redis or Memcached) outlets information in memory for speedy accessibility.
two. Consumer-facet caching (like browser caching or CDN caching) shops static files near to the person.
Caching decreases databases load, improves pace, and tends to make your application more productive.
Use caching for things which don’t modify normally. And usually ensure that your cache is updated when details does modify.
To put it briefly, load balancing and caching are easy but highly effective tools. Collectively, they assist your app manage additional customers, remain rapid, and recover from difficulties. If you propose to grow, you require both.
Use Cloud and Container Resources
To develop scalable purposes, you need resources that allow your application mature easily. That’s in which cloud platforms and containers can be found in. They offer you adaptability, reduce setup time, and make scaling Significantly smoother.
Cloud platforms like Amazon World wide web Services (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and companies as you require them. You don’t really have to buy components or guess future capacity. When visitors raises, you'll be able to incorporate far more methods with just a couple clicks or mechanically using auto-scaling. When traffic drops, you are able to scale down to save money.
These platforms also offer services like managed databases, storage, load balancing, and security applications. You could deal with setting up your application as an alternative to controlling infrastructure.
Containers are Yet another crucial Instrument. A container offers your application and all the things it really should operate—code, libraries, options—into 1 device. This can make it effortless to move your application involving environments, out of your laptop to the cloud, without the need of surprises. Docker is the most well-liked Instrument for this.
Once your application makes use of numerous containers, resources like Kubernetes help you take care of them. Kubernetes handles deployment, scaling, and recovery. If one aspect of the app crashes, it restarts it mechanically.
Containers also ensure it is easy to individual elements of your application into providers. You can update or scale sections independently, which can be perfect for functionality and reliability.
Briefly, employing cloud and container tools suggests you are able to scale rapid, deploy very easily, and Get better rapidly when complications take place. If you prefer your app to improve with out boundaries, start employing these applications early. They conserve time, lower danger, and allow you to remain centered on setting up, not fixing.
Keep an eye on All the things
Should you don’t watch your software, you won’t know when items go Erroneous. Checking assists you see how your application is accomplishing, spot concerns early, and make superior conclusions as your app grows. It’s a important Section of building scalable techniques.
Start off by monitoring essential metrics like CPU usage, memory, disk space, and response time. These let you know how your servers and companies are executing. Applications like Prometheus, Grafana, Datadog, or New Relic can help you gather and visualize this info.
Don’t just keep an eye on your servers—keep an eye on your application far too. Regulate how much time it's going to take for users to load pages, how frequently mistakes take place, and the place more info they arise. Logging equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring inside your code.
Create alerts for crucial troubles. By way of example, When your response time goes over a limit or a service goes down, you should get notified immediately. This allows you take care of difficulties rapid, typically just before consumers even observe.
Monitoring can also be useful after you make improvements. In case you deploy a fresh function and find out a spike in glitches or slowdowns, you'll be able to roll it back right before it brings about actual damage.
As your application grows, site visitors and information maximize. With no monitoring, you’ll pass up signs of trouble until eventually it’s also late. But with the correct tools in position, you stay on top of things.
In brief, checking aids you keep the app responsible and scalable. It’s not almost recognizing failures—it’s about comprehension your system and making certain it works properly, even under pressure.
Ultimate Thoughts
Scalability isn’t just for significant organizations. Even compact apps have to have a powerful Basis. By designing meticulously, optimizing wisely, and utilizing the right instruments, you can Create applications that develop efficiently without breaking under pressure. Start out small, Consider significant, and Develop sensible.